A shell script is just a text file, with .sh extension, having executable permission. A shell can read this file and act on the commands. shell also provide different programming features to make the script truly powerful.
We can create a shell script using the following steps:
- create a file using Gedit or VI editor and name it with extension .sh or .bash
- Start the script with #!/bin/sh or #!/bin/bash
- Write some codes.
- Save the script file as filename.sh or filename.bash
- For executing the script inside terminal, type bash filename.sh
Clear
echo “hello world”
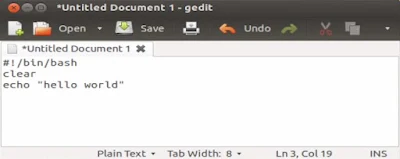
Now we can save above shell script, using some filename with extension .sh or .bash
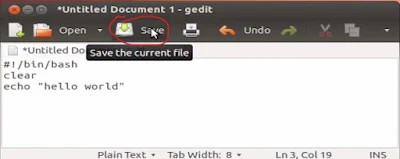
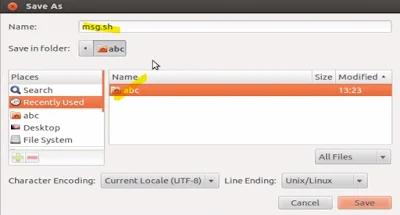
E.g. msg.sh
Now to run this script in the terminal we can use bash msg.sh command.


Comments
Comments are information for a user not for a computer or shell. While executing a shell program, comments are always ignored. We can add comments in a shell program using # symbol.
E.g.
#!/bin/bash
echo “hello world” #this will produce "hello world" message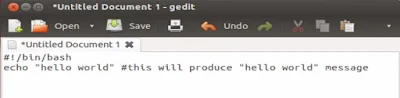
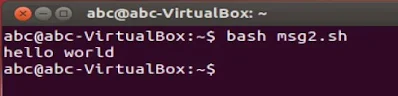
Variables are termed as container and we can place data into this containers and then we can use these containers data by simply naming the container
We can declare shell variable using following syntax:
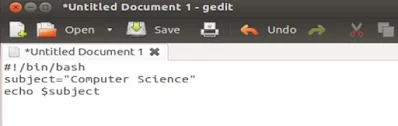
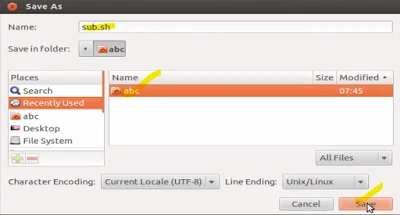
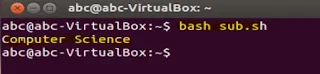
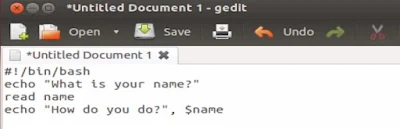
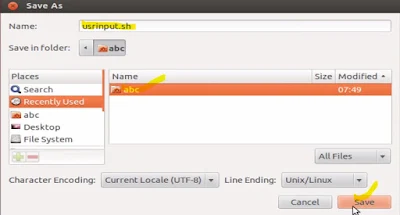
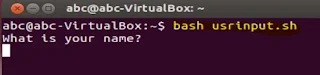
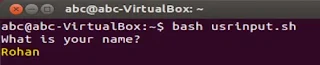
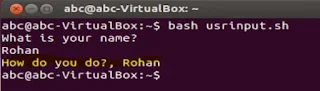
<variablename> = “value”
E.g. variablename = “Hello”
Now we can use/access variable’s value by writing variable name using following syntax:
$<variablename>
$<variablename>
E.g.
#!/bin/bash
subject = "Computer Science"
echo $subject
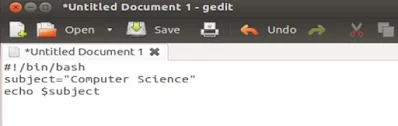
Now we can save this file
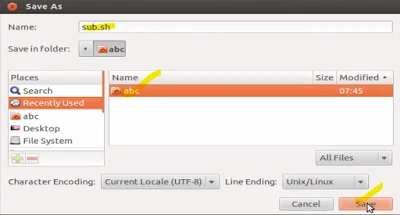
To run this file we need to use bash command with file name i.e. sub.sh
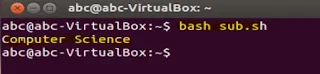
Also we can get input from user using read command along with variable name
E.g. read <variablename>
E.g. read <variablename>
E.g.
#!/bin/bash
echo "What is your name?"
read name
echo "How do you do?", $name
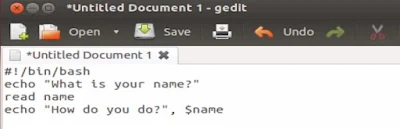
Now we can save this file
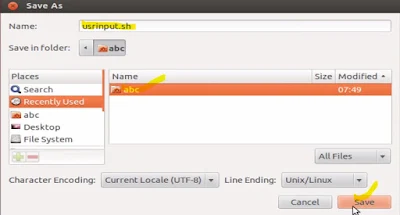
To run this file we need to use bash command with file name i.e. usrinput.sh
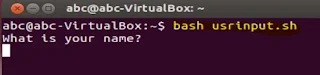
Now we need to enter input
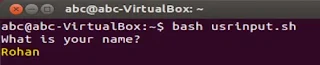
After input, we will get an output with a string message How do you do? including user input i.e. Rohan
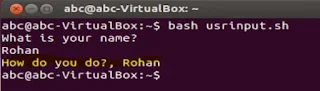